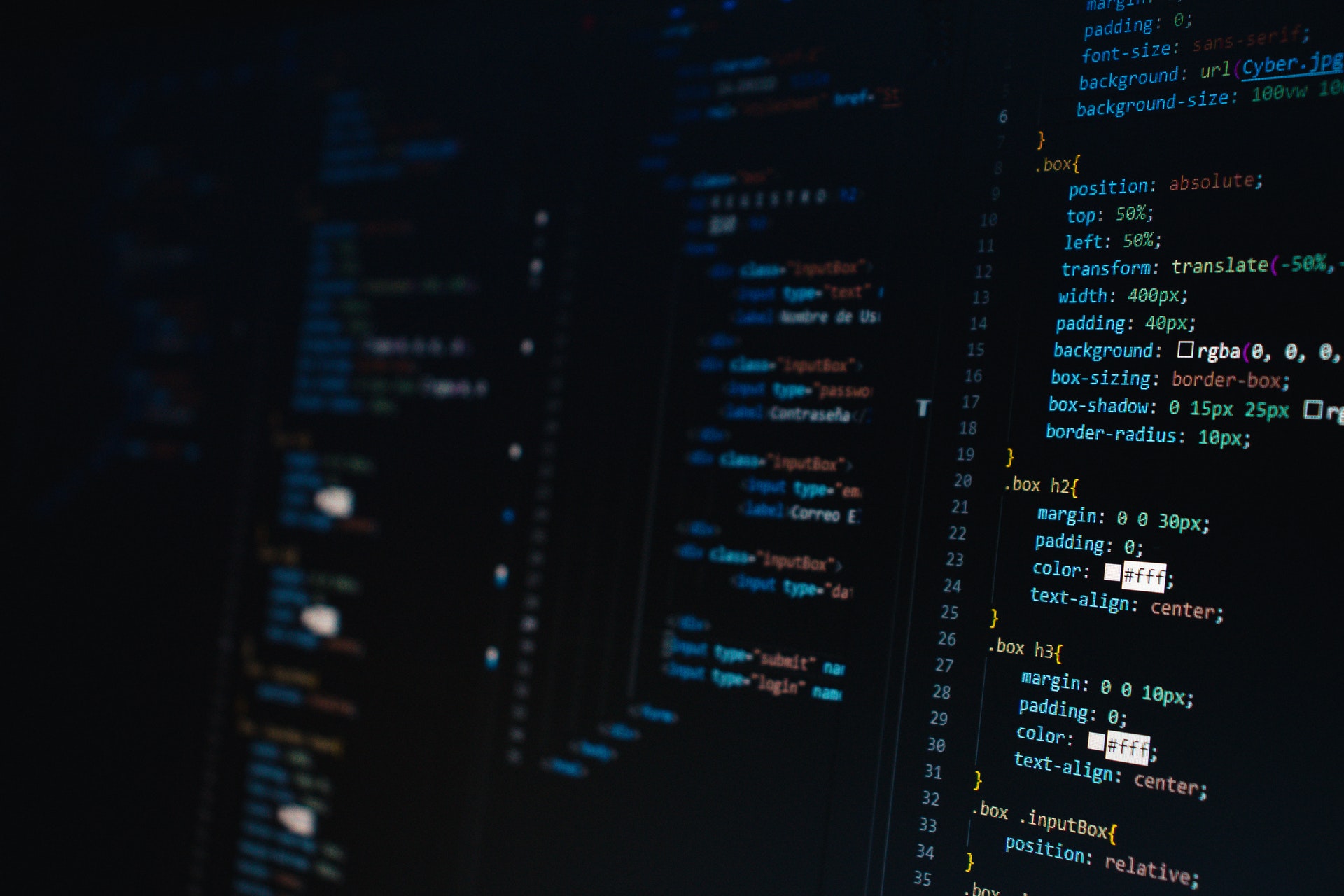
The road to clean code
Introduction.
This post was written as a guideline for writing better code; bookmark it so you can easily refer back to it.... as we all know, clean coding is not a skill that can be learned overnight.
Writing clear, comprehensible, and maintainable code is a skill that every developer should have. In this article, we'll look at some of the best practices for improving code quality, by writing better functions, classes and modules, and comments, as well as providing code samples for some of these concepts.
Why Write Clean Code ?
Writing clean code is important because it allows you to clearly communicate with the next person who works with what you've written. The more declarative code is the better it communicates, and the easier it is to fix problems when they arise. - Echobind
- Easier for you and others to understand.
- Clean code is also maintainable.
- Other
Why should you read this article all the way through?
:)
File Structure.
A successful file structure organizes your data and code with the goal of repeatability, making it easier for you and your collaborators to revisit, revise and develop your project. File structures are not fixed entities, but rather build a framework that communicates the function and purpose of elements within a project by separating concerns into a hierarchy of folders and using consistent, chronological, and descriptive names. - George Sun
- One language per file.
- Avoid generating another language with another, e.g. writing xml or html using TS or C# strings.
- Consider using relative file paths, not absolute paths, to facilitate shareability.
- Consistency is key
Functions || Methods.
"If you can extract a function out of your functions, then your function is doing more than two things. rather have bunch of functions with good names". Uncle Bob
Guidelines
- Functions should do one thing. They should do it well. They should do it only. – Clean Code
- Naming guideline, use "verbs" (action words)
- The method/function name says it all.
- Avoid functions with more than 3 levels of indentation.
- Avoid passing more than 3 variables as arguments, if 4+ that may just be a class.
- Avoid passing booleans into functions, that means there must be an if statement
Validating Function Arguments
Add guard clauses to fail fast if the input is invalid as they help seperate validation from actual business rules later on in the code. e.g.
function doSomething(price: number) {
if(price <= 0)
throw new ArgumentException("price must be greater than 0")
// do something
}
- Try return early in functions.
Classes and Interfaces.
Naming
- Class name guidelines, (Nouns, Single responsibility)
- Use Pascal Case for class names
- Start Interface names with I, followed by a meaningful name in Pascal case
Variables
- Strive to create "Mayfly variables". Declare variables close to first use. not at the top all the time. - Uncle Bob
- Remove variables from scope as soon as possible.
Other
- Classes over 100 lines should be thought through, for possible "separation of concerns"
Comments.
"A comment is a failure to express yourself in code. If you fail, then write a comment; but try not to fail." Uncle Bob Martin, emphasis on in-house code.
Self documenting code uses well chosen variable names (and function names) to make the code read as close to English as possible. This should be your goal.
No Need to a Comment Here
// saving user
function saveUser(user: User) {
}
- Clear Dead Code or Zombie code, double check if its safe to delete.
- Dot not leave code in comments: Please, Do Not. This one is serious because others who see the code will be afraid to delete it because they do not know if it is there for a reason.
Clean Code Principles.
There are three core principles to remember writing clean code:
- Choose the right tool for the job
- Optimize the signal-to-noise ratio
- Strive to write self-documenting code
Other Principles:
- Refactor as soon as possible
- Coding Standards/Conventions – So we don't end up having different code with different coding styles among our team.
- SOLID – Design principles, this is a topic in itself but mastering the S will already help us in a tremendous way.
- Design Patterns – There are tons of design patterns out there, some people already resolved our problem.
Bonus.
"Its not easy to write an algorithm, but once it works, your not done its a sign to clean your code." - Uncle Bob
"Clean code is simple and direct. Clean code reads like well-written prose. Clean code never obscures the designer’s intent but rather is full of crisp abstractions and straightforward lines of control. "- Grady Booch author of Object Oriented Analysis and Design with Applications
Try reading your code Aload, it exposes problems.
Reading code is harder than writing.
"If you can't write unit tests for your code, you prob doing something dodgy." Vhlnd.
Always design first, then code. Take time to understand the requirement, discuss with the team, design properly, and then start coding. Do not touch the code until the whole thing has been designed at low level.
Conclusion.

Sources
https://mitcommlab.mit.edu/broad/commkit/file-structure/ https://blog.echobind.com/why-should-i-write-clean-code-6068548dbd7e https://www.pluralsight.com/blog/software-development/7-reasons-clean-code-matters https://arghya.xyz/articles/coding-guidelineshttps://www.arcanys.com/blog/the-importance-of-clean-code
Our greatest weakness lies in giving up. The most certain way to succeed is always to try just one more time.